//Superhero Power Plant
//fades all pixels subtly
//code by Tony Sherwood for Adafruit Industries
#include <Adafruit_NeoPixel.h>
#define PIN 1
// Parameter 1 = number of pixels in strip
// Parameter 2 = pin number (most are valid)
// Parameter 3 = pixel type flags, add together as needed:
// NEO_KHZ800 800 KHz bitstream (most NeoPixel products w/WS2812 LEDs)
// NEO_KHZ400 400 KHz (classic 'v1' (not v2) FLORA pixels, WS2811 drivers)
// NEO_GRB Pixels are wired for GRB bitstream (most NeoPixel products)
// NEO_RGB Pixels are wired for RGB bitstream (v1 FLORA pixels, not v2)
Adafruit_NeoPixel strip = Adafruit_NeoPixel(17, PIN, NEO_GRB + NEO_KHZ800);
int alpha; // Current value of the pixels
int dir = 1; // Direction of the pixels... 1 = getting brighter, 0 = getting dimmer
int flip; // Randomly flip the direction every once in a while
int minAlpha = 25; // Min value of brightness
int maxAlpha = 100; // Max value of brightness
int alphaDelta = 5; // Delta of brightness between times through the loop
void setup() {
strip.begin();
strip.show(); // Initialize all pixels to 'off'
}
void loop() {
flip = random(32);
if(flip > 20) {
dir = 1 - dir;
}
// Some example procedures showing how to display to the pixels:
if (dir == 1) {
alpha += alphaDelta;
}
if (dir == 0) {
alpha -= alphaDelta;
}
if (alpha < minAlpha) {
alpha = minAlpha;
dir = 1;
}
if (alpha > maxAlpha) {
alpha = maxAlpha;
dir = 0;
}
// Change the line below to alter the color of the lights
// The numbers represent the Red, Green, and Blue values
// of the lights, as a value between 0(off) and 1(max brightness)
//
// EX:
// colorWipe(strip.Color(alpha, 0, alpha/2)); // Pink
colorWipe(strip.Color(0, 0, alpha)); // Blue
}
// Fill the dots one after the other with a color
void colorWipe(uint32_t c) {
for(uint16_t i=0; i<strip.numPixels(); i++) {
strip.setPixelColor(i, c);
strip.show();
}
}
Iron Man Arc Reactor
A cheap replica of the famed chest-piece of Tony Stark, AKA Iron Man.
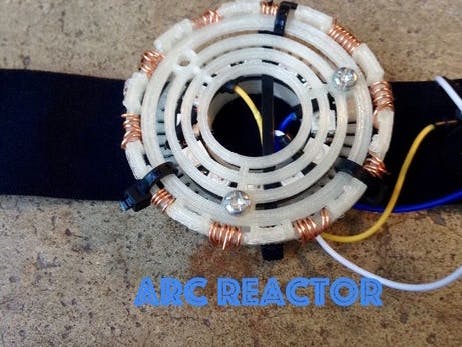
Comments